Visual Basic (Declaration) | |
---|---|
Public Property FeatureLayer As FeatureLayer |
C# | |
---|---|
public FeatureLayer FeatureLayer {get; set;} |
How to use:
Click the button to add the FeatureLayer to the Map Control and wire-up an AttachmentEditor if applicable. The code example demonstrates adding a FeatureLayer dynamically at run-time. If the FeatureLayer has attachments enabled (i.e. True) in the FeatureService, dynamically add the functionality to associate an AttachmentEditor with the FeatureLayer for uploading/downloading/deleting attachments associated with the Graphic features in the FeatureLayer.
The XAML code in this example is used in conjunction with the code-behind (C# or VB.NET) to demonstrate the functionality.
The following screen shot corresponds to the code example in this page.
XAML | ![]() |
---|---|
<!-- This code example demonstrates adding a FeatureLayer dynamically at run-time. If the FeatureLayer has attachments enabled (i.e. True) in the FeatureService, dynamically add the functionality to associate an AttachmentEditor with the FeatureLayer for uploading/downloading/deleting attachments associated with the Graphic features in the FeatureLayer. --> <Grid x:Name="LayoutRoot"> <!-- Add a button with instructions. The Click Event is enabled to for code-behind functionality. --> <Button Content="Add the FeatureLayer to the Map and wire-up an AttachmentEditor if applicable." Height="23" HorizontalAlignment="Left" Margin="12,6,0,0" Name="Button1" VerticalAlignment="Top" Width="616" Click="Button1_Click"/> <!-- Add a Label telling the user to supply a FeatureLayer.Url --> <sdk:Label Height="28" HorizontalAlignment="Left" Name="Label1" VerticalAlignment="Top" Width="182" Content="Enter a Url for a FeatureLayer:" Margin="12,41,0,0" /> <!-- Add a default text string for a FeatureLayer.Url where attachements are enabled in the FeatureServer. A FeatureLayer.Url WITH Attachments: "http://sampleserver3.arcgisonline.com/ArcGIS/rest/services/SanFrancisco/311Incidents/FeatureServer/0" A FeatureLayer.Url WITHOUT Attachments: "http://sampleserver3.arcgisonline.com/ArcGIS/rest/services/Fire/Sheep/FeatureServer/0" --> <TextBox Height="23" HorizontalAlignment="Left" Margin="12,58,0,0" Name="TextBox_FeatureLayerUrl" VerticalAlignment="Top" Width="628" Text="http://sampleserver3.arcgisonline.com/ArcGIS/rest/services/SanFrancisco/311Incidents/FeatureServer/0"/> <!-- Add a Label informing the user if FeatureLayer supports attachents. --> <sdk:Label Height="28" HorizontalAlignment="Left" Margin="12,98,0,0" Name="Label2" VerticalAlignment="Top" Width="300" Content="Does the FeatureLayer support having attachments?"/> <!-- This is the TexBlock that will provide a True or False answer. A True means the FeatureLayer supports having attachments. A False means the FeatureLayer does not support adding attachments. The TextBlock is invisible by default until the user clicks the Button1. --> <TextBlock Height="23" HorizontalAlignment="Left" Margin="307,98,0,0" Name="TextBlock_FeatureLayerLayerInfoHasAttachments" Text="TextBlock" VerticalAlignment="Top" Visibility="Collapsed"/> <!-- Add an ESRI Map Control. --> <esri:Map Background="White" HorizontalAlignment="Left" Margin="12,124,0,0" Name="Map1" VerticalAlignment="Top" Height="344" Width="320"> <!-- Add a backdrop ArcGISTiledMapServiceLayer. --> <esri:ArcGISTiledMapServiceLayer Url="http://services.arcgisonline.com/ArcGIS/rest/services/World_Street_Map/MapServer" /> </esri:Map> <!-- Add a default AttachmentEditor Control and set it to be invisible by default. Only if the supplied FeatureLayer has attachments = True will it be displayed. --> <esri:AttachmentEditor HorizontalAlignment="Left" Margin="338,124,0,0" Name="AttachmentEditor1" VerticalAlignment="Top" Height="344" Width="290" Visibility="Collapsed"/> </Grid> |
C# | ![]() |
---|---|
private void Button1_Click(object sender, System.Windows.RoutedEventArgs e) { // This function is the main driver for the sample code. It creates a FeatureLayer dynamically // and adds it to the Map Control. The FeatureLayer.Initialized Event handler is dynamically // wired-up and activated when the FeatureLayer is added to the Map. // Create a new FeatureLayer using the Url text string supplied by the user. ESRI.ArcGIS.Client.FeatureLayer myFeatureLayer = new ESRI.ArcGIS.Client.FeatureLayer(); myFeatureLayer.Url = TextBox_FeatureLayerUrl.Text; // Add the FeatureLayer to the Map. Map1.Layers.Add(myFeatureLayer); // Create the FeatureLayer.Intialized Event handler. It will be invoked automatically // when the FeatureLayer is added to the Map Control. myFeatureLayer.Initialized += myFeatureLayer_Initialized; } private void myFeatureLayer_Initialized(object sender, EventArgs e) { // This function executes as a result of the FeatureLayer being initialized for the first time. // If FeatureLayer suppports having attachments in the FeatureService, set the properties on // the AttachmentEditor Control and wire-up the FeatureLayer.MouseLeftButtonUp Event handler // which will allow users to click on a Graphic and display any attachment information. // Obtain the FeatureLayer from the sender argument. ESRI.ArcGIS.Client.FeatureLayer myFeatureLayer = sender as ESRI.ArcGIS.Client.FeatureLayer; // Get a FeatureService.FeatureLayerInfo object from the FeatureLayer. ESRI.ArcGIS.Client.FeatureService.FeatureLayerInfo myFeatureLayerInfo = myFeatureLayer.LayerInfo; // Display if attachments have been enabled in the TextBlock. TextBlock_FeatureLayerLayerInfoHasAttachments.Text = myFeatureLayerInfo.HasAttachments.ToString(); TextBlock_FeatureLayerLayerInfoHasAttachments.Visibility = Windows.Visibility.Visible; if (myFeatureLayerInfo.HasAttachments == true) { // If attachments are enabled in the FeatureLayer's FeatureService, set up the properties // of the AttachmentEditor. AttachmentEditor1.FeatureLayer = myFeatureLayer; AttachmentEditor1.Filter = "Image Files|*.jpg;*.gif;*.png;*.bmp|Adobe PDF Files (.pdf)|*.pdf"; AttachmentEditor1.FilterIndex = 1; AttachmentEditor1.Multiselect = true; AttachmentEditor1.Visibility = Windows.Visibility.Visible; // Add the FeatureLayer.MouseLeftButtonUp Event handler to allow users to click on a // Graphic and display any attachment information. myFeatureLayer.MouseLeftButtonUp += myFeatureLayer_MouseLeftButtonUp; } } private void myFeatureLayer_MouseLeftButtonUp(object sender, ESRI.ArcGIS.Client.GraphicMouseButtonEventArgs e) { // This function obtains the FeatureLayer, loops through all of the Graphic features and // un-selects them. Then for the Graphic for which the user clicked with the mouse select // it and use that Graphic to set the AttachmentEditor.GraphicSource to enable uploading, // downloading, and deleting attachment files on the ArcGIS Server backend database. // Get the FeatureLayer from the sender object of the FeatureLayer.MouseLeftButtonUp Event. ESRI.ArcGIS.Client.FeatureLayer myFeatureLayer = sender as ESRI.ArcGIS.Client.FeatureLayer; // Loop through all of the Graphics in the FeatureLayer and UnSelect them. for (int i = 0; i < myFeatureLayer.SelectionCount; i++) { myFeatureLayer.SelectedGraphics.ToList()[i].UnSelect(); } // Select the Graphic from the e object which will change visibly on the Map via highlighting. e.Graphic.Select(); // Setting the AttachmentEditor.GraphicSource immediately causes the AttachmentEditor Control // to update with any attachments currently associated with the Graphic. AttachmentEditor1.GraphicSource = e.Graphic; } |
VB.NET | ![]() |
---|---|
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.Windows.RoutedEventArgs) ' This function is the main driver for the sample code. It creates a FeatureLayer dynamically ' and adds it to the Map Control. The FeatureLayer.Initialized Event handler is dynamically ' wired-up and activated when the FeatureLayer is added to the Map. ' Create a new FeatureLayer using the Url text string supplied by the user. Dim myFeatureLayer As New ESRI.ArcGIS.Client.FeatureLayer myFeatureLayer.Url = TextBox_FeatureLayerUrl.Text ' Add the FeatureLayer to the Map. Map1.Layers.Add(myFeatureLayer) ' Create the FeatureLayer.Intialized Event handler. It will be invoked automatically ' when the FeatureLayer is added to the Map Control. AddHandler myFeatureLayer.Initialized, AddressOf myFeatureLayer_Initialized End Sub Private Sub myFeatureLayer_Initialized(ByVal sender As Object, ByVal e As EventArgs) ' This function executes as a result of the FeatureLayer being initialized for the first time. ' If FeatureLayer suppports having attachments in the FeatureService, set the properties on ' the AttachmentEditor Control and wire-up the FeatureLayer.MouseLeftButtonUp Event handler ' which will allow users to click on a Graphic and display any attachment information. ' Obtain the FeatureLayer from the sender argument. Dim myFeatureLayer As ESRI.ArcGIS.Client.FeatureLayer = TryCast(sender, ESRI.ArcGIS.Client.FeatureLayer) ' Get a FeatureService.FeatureLayerInfo object from the FeatureLayer. Dim myFeatureLayerInfo As ESRI.ArcGIS.Client.FeatureService.FeatureLayerInfo = myFeatureLayer.LayerInfo ' Display if attachments have been enabled in the TextBlock. TextBlock_FeatureLayerLayerInfoHasAttachments.Text = myFeatureLayerInfo.HasAttachments.ToString TextBlock_FeatureLayerLayerInfoHasAttachments.Visibility = Windows.Visibility.Visible If myFeatureLayerInfo.HasAttachments = True Then ' If attachments are enabled in the FeatureLayer's FeatureService, set up the properties ' of the AttachmentEditor. AttachmentEditor1.FeatureLayer = myFeatureLayer AttachmentEditor1.Filter = "Image Files|*.jpg;*.gif;*.png;*.bmp|Adobe PDF Files (.pdf)|*.pdf" AttachmentEditor1.FilterIndex = 1 AttachmentEditor1.Multiselect = True AttachmentEditor1.Visibility = Windows.Visibility.Visible ' Add the FeatureLayer.MouseLeftButtonUp Event handler to allow users to click on a ' Graphic and display any attachment information. AddHandler myFeatureLayer.MouseLeftButtonUp, AddressOf myFeatureLayer_MouseLeftButtonUp End If End Sub Private Sub myFeatureLayer_MouseLeftButtonUp(ByVal sender As Object, ByVal e As ESRI.ArcGIS.Client.GraphicMouseButtonEventArgs) ' This function obtains the FeatureLayer, loops through all of the Graphic features and ' un-selects them. Then for the Graphic for which the user clicked with the mouse select ' it and use that Graphic to set the AttachmentEditor.GraphicSource to enable uploading, ' downloading, and deleting attachment files on the ArcGIS Server backend database. ' Get the FeatureLayer from the sender object of the FeatureLayer.MouseLeftButtonUp Event. Dim myFeatureLayer As ESRI.ArcGIS.Client.FeatureLayer = TryCast(sender, ESRI.ArcGIS.Client.FeatureLayer) ' Loop through all of the Graphics in the FeatureLayer and UnSelect them. For i As Integer = 0 To myFeatureLayer.SelectionCount - 1 myFeatureLayer.SelectedGraphics.ToList()(i).UnSelect() Next i ' Select the Graphic from the e object which will change visibly on the Map via highlighting. e.Graphic.Select() ' Setting the AttachmentEditor.GraphicSource immediately causes the AttachmentEditor Control ' to update with any attachments currently associated with the Graphic. AttachmentEditor1.GraphicSource = e.Graphic End Sub |
To use the AttachmentEditor Control the AttachmentEditor.FeatureLayer Property must be set to a valid FeatureLayer that has attachments enabled in an ArcGIS Server FeatureServer. Developers who do not have direct access to management of ArcGIS Server but need to know if An ArcGIS Server FeatureLayer has attachments enabled can do so programmatically by using the FeatureLayer.LayerInfo Property to return an ESRI.ArcGIS.Client.FeatureService.FeatureLayerInfo object. Use the ESRI.ArcGIS.Client.FeatureService.FeatureLayerInfo.HasAttachments Property to obtain a Boolean value to know if attachments are enabled for the FeatureService. A code example is provided in this document for programmatically discovering if a FeatureLayer Has Attachments.
Alternatively, developers can specify the FeatureLayer.Url in an web browser and look for the Has Attachments section to see if the value is True, see the following screen shot:
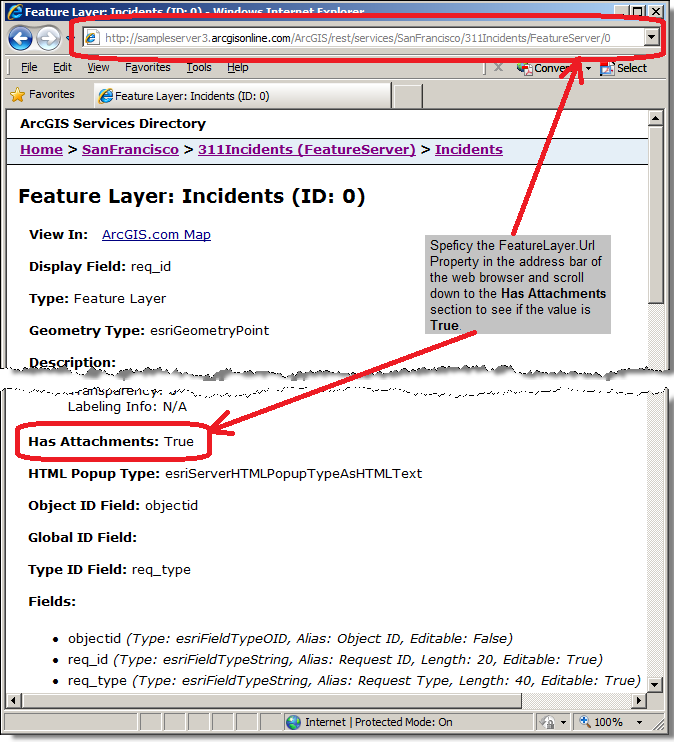
If the AttachmentEditor.FeatureLayer Property is not set to a valid ArcGIS Server FeatureServer service with attachments enabled, a Visual Studio NotSupportedException error containing syntax similar to the following will occur: "Layer does not support attachments" or "Layer does not support adding attachments". It should be noted the FeatureLayer.Url Property of an ArcGIS Server MapServer service looks very similar to FeatureServer and might even display a Has Attributes value equal True when viewed in a web browser but this should not be used for the AttachmentEditor Control. A valid FeatureServer will have the words “FeatureServer” in the URL, not “MapServer” which will cause errors.
Target Platforms: Windows XP Professional, Windows Server 2003 family, Windows Vista, Windows Server 2008 family