
Visual Basic (Declaration) | |
---|---|
Public Class AttachmentEditor Inherits System.Windows.Controls.Control |
C# | |
---|---|
public class AttachmentEditor : System.Windows.Controls.Control |
How to use:
When the Map loads click on a point feature in the FeatureLayer to select the Graphic. Then use the AttachmentEditor to add, delete, and download files.
The XAML code in this example is used in conjunction with the code-behind (C# or VB.NET) to demonstrate the functionality.
The following screen shot corresponds to the code example in this page.
XAML | ![]() |
---|---|
<Grid x:Name="LayoutRoot"> <!-- Provide the instructions on how to use the sample code. --> <TextBlock Height="64" HorizontalAlignment="Left" Name="TextBlock1" VerticalAlignment="Top" Width="512" TextWrapping="Wrap" Margin="12,12,0,0" Text="When the Map loads click on a point feature in the FeatureLayer to select the Graphic. Then use the AttachmentEditor to add, delete, and download files." /> <!-- Add a Map control with an ArcGISTiledMapeServiceLayer and a FeatureLayer. It is important to provide a Name for the Map Control as this will be used by the AttachmentEditor Control. Define and initial Extent for the map. --> <esri:Map Background="White" HorizontalAlignment="Left" Margin="12,100,0,0" Name="MyMap" VerticalAlignment="Top" Height="272" Width="400" Extent="-13625087,4547888,-13623976,4548643"> <!-- Add a backdrop ArcGISTiledMapServiceLayer. --> <esri:ArcGISTiledMapServiceLayer Url="http://services.arcgisonline.com/ArcGIS/rest/services/World_Street_Map/MapServer" /> <!-- The FeatureLayer is based upon an ArcGIS Server 'FeatureServer' service not a 'MapServer' service. It is important to provide an ID value as this will be used for Binding in the AttachementEditor. The MouseLeftButtonUp Event handler has been defined for the FeatureLayer. --> <esri:FeatureLayer ID="IncidentsLayer" Url="http://sampleserver3.arcgisonline.com/ArcGIS/rest/services/SanFrancisco/311Incidents/FeatureServer/0" MouseLeftButtonUp="FeatureLayer_MouseLeftButtonUp" AutoSave="False" DisableClientCaching="True" OutFields="*" Mode="OnDemand" /> </esri:Map> <!-- Wrap the Attachment Editor inside of a Grid and other controls to provide a stylistic look and to provide instructions to the user. --> <Grid HorizontalAlignment="Left" VerticalAlignment="Top" Margin="450,100,15,0" > <Rectangle Fill="BurlyWood" Stroke="Green" RadiusX="15" RadiusY="15" Margin="0,0,0,5" > <Rectangle.Effect> <DropShadowEffect/> </Rectangle.Effect> </Rectangle> <Rectangle Fill="#FFFFFFFF" Stroke="Aqua" RadiusX="5" RadiusY="5" Margin="10,10,10,15" /> <StackPanel Orientation="Vertical" HorizontalAlignment="Left"> <TextBlock Text="Click on a point feature to select it, and click the 'Add' button to attach a file." Width="275" TextAlignment="Left" Margin="20,20,20,5" TextWrapping="Wrap" FontWeight="Bold"/> <!-- Add an AttachmentEditor. Provide a x:Name for the AttachmentEditor so that it can be used in the code-behind file. The FeatureLayer Property is bound to the 'IncidentLayer' of the Map Control. Two groups of Filter types are used (you can have multiple file extensions in a group) to drive the OpenFileDialog. The FilterIndex shows the first group of Filter Types in the OpenFileDialog dropdown list. Only allow the user to upload (i.e. Add) one file at a time using the OpenFileDialog with the Multiselect set to False. An UploadFailed Event has been defined for the code-behind. --> <esri:AttachmentEditor x:Name="MyAttachmentEditor" VerticalAlignment="Top" Margin="20,5,20,20" Background="LightGoldenrodYellow" Width="280" Height="190" HorizontalAlignment="Right" FeatureLayer="{Binding Layers[IncidentsLayer], ElementName=MyMap}" Filter="Image Files|*.jpg;*.gif;*.png;*.bmp|Adobe PDF Files (.pdf)|*.pdf" FilterIndex="1" Multiselect="False" UploadFailed="MyAttachmentEditor_UploadFailed"> </esri:AttachmentEditor> </StackPanel> </Grid> </Grid> |
C# | ![]() |
---|---|
private void FeatureLayer_MouseLeftButtonUp(object sender, ESRI.ArcGIS.Client.GraphicMouseButtonEventArgs e) { // This function obtains the FeatureLayer, loops through all of the Graphic features and // un-selects them. Then for the Graphic for which the user clicked with the mouse select // it and use that Graphic to set the AttachmentEditor.GraphicSource to enable uploading, // downloading, and deleting attachment files on the ArcGIS Server backend database. // Get the FeatureLayer from the sender object of the FeatureLayer.MouseLeftButtonUp Event. ESRI.ArcGIS.Client.FeatureLayer myFeatureLayer = sender as ESRI.ArcGIS.Client.FeatureLayer; // Loop through all of the Graphics in the FeatureLayer and UnSelect them. for (int i = 0; i < myFeatureLayer.SelectionCount; i++) { myFeatureLayer.SelectedGraphics.ToList()[i].UnSelect(); } // Select the Graphic from the e object which will change visibly on the Map via highlighting. e.Graphic.Select(); // Setting the AttachmentEditor.GraphicSource immediately causes the AttachmentEditor Control // to update with any attachments currently associated with the Graphic. MyAttachmentEditor.GraphicSource = e.Graphic; } private void MyAttachmentEditor_UploadFailed(object sender, ESRI.ArcGIS.Client.Toolkit.AttachmentEditor.UploadFailedEventArgs e) { // Display any error messages that may occur if the AttachmentEditor fails to upload a file. MessageBox.Show(e.Result.Message); } |
VB.NET | ![]() |
---|---|
Private Sub FeatureLayer_MouseLeftButtonUp(ByVal sender As Object, ByVal e As ESRI.ArcGIS.Client.GraphicMouseButtonEventArgs) ' This function obtains the FeatureLayer, loops through all of the Graphic features and ' un-selects them. Then for the Graphic for which the user clicked with the mouse select ' it and use that Graphic to set the AttachmentEditor.GraphicSource to enable uploading, ' downloading, and deleting attachment files on the ArcGIS Server backend database. ' Get the FeatureLayer from the sender object of the FeatureLayer.MouseLeftButtonUp Event. Dim myFeatureLayer As ESRI.ArcGIS.Client.FeatureLayer = TryCast(sender, ESRI.ArcGIS.Client.FeatureLayer) ' Loop through all of the Graphics in the FeatureLayer and UnSelect them. For i As Integer = 0 To myFeatureLayer.SelectionCount - 1 myFeatureLayer.SelectedGraphics.ToList()(i).UnSelect() Next i ' Select the Graphic from the e object which will change visibly on the Map via highlighting. e.Graphic.Select() ' Setting the AttachmentEditor.GraphicSource immediately causes the AttachmentEditor Control ' to update with any attachments currently associated with the Graphic. MyAttachmentEditor.GraphicSource = e.Graphic End Sub Private Sub MyAttachmentEditor_UploadFailed(ByVal sender As Object, ByVal e As ESRI.ArcGIS.Client.Toolkit.AttachmentEditor.UploadFailedEventArgs) ' Display any error messages that may occur if the AttachmentEditor fails to upload a file. MessageBox.Show(e.Result.Message) End Sub |
The AttachmentEditor is a User Interface (UI) control that allows clients to upload, download, and delete files associated with Graphic features in a FeatureLayer of a backend ArcGIS Server FeatureServer database. The AttachmentEditor Control can be created in at design time in XAML or in dynamically at runtime in the code-behind. The AttachmentEditor Control is one of several controls available in the Toolbox in Visual Studio when the ESRI ArcGIS API for Silverlight/WPF is installed, see the following screen shot:
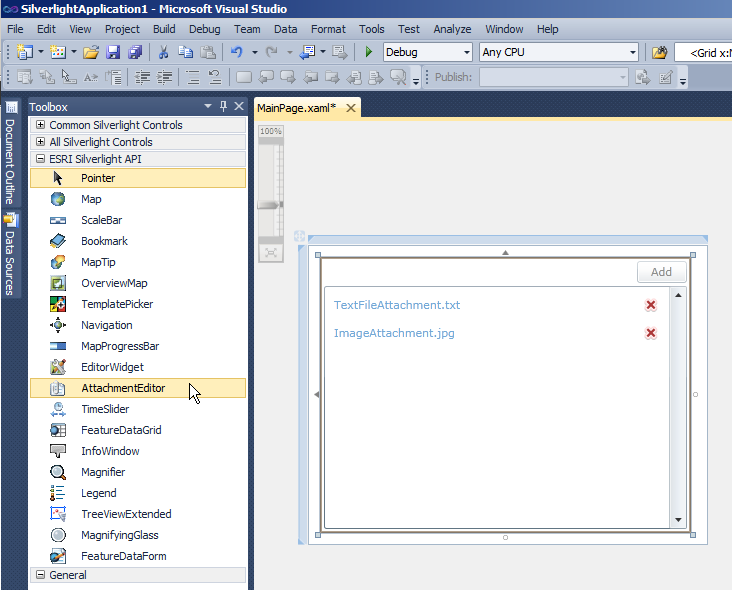
The default appearance of the AttachmentEditor Control can be modified using numerous inherited Properties from System.Windows.FrameworkElement, System.Windows.UIElement, and System.Windows.Controls. An example of some of these Properties include: .Height, .Width, .BackGround, .BorderBrush, .BorderThickness, .FontFamily, .FontSize, .FontStretch, .FontStyle, .Foreground, .HorizontalAlignment, .VerticalAlignment, .Margin, .Opacity, .Visibility, etc.
Note: you cannot change the core behavior of the sub-components (i.e. Button, ListBox, etc.) of the AttachmentEditor Control using standard Properties or Methods alone. To change the core behavior of the sub-components and their appearance of the Control, developers can modify the Control Template in XAML and the associated code-behind file. The easiest way to modify the UI sub-components is using Microsoft Expression Blend. Then developers can delete/modify existing or add new sub-components in Visual Studio to create a truly customized experience. A general approach to customizing a Control Template is discussed in the ESRI blog entitled: Use control templates to customize the look and feel of ArcGIS controls.
Additionally, changing the default behavior (or functionality) and appearance of the ListBox sub-component that displays information about attachments in the AttachmentEditor Control can be modified using a DataTemplate via the AttachmentEditor.ItemTemplate Property and writing you own custom code when necessary. When defining the DataTemplate, the Binding Properties that developers want to use to control the behavior of the AttachmentEditor are based upon those in the ESRI.ArcGIS.Client.FeatureService.AttachmentInfo Class.
To use the AttachmentEditor Control the AttachmentEditor.FeatureLayer Property must be set to a valid FeatureLayer that has attachments enabled in an ArcGIS Server FeatureServer. Developers who do not have direct access to management of ArcGIS Server but need to know if An ArcGIS Server FeatureLayer has attachments enabled can specify the FeatureLayer.Url in an web browser and look for the Has Attachments section to see if the value is True, see the following screen shot:
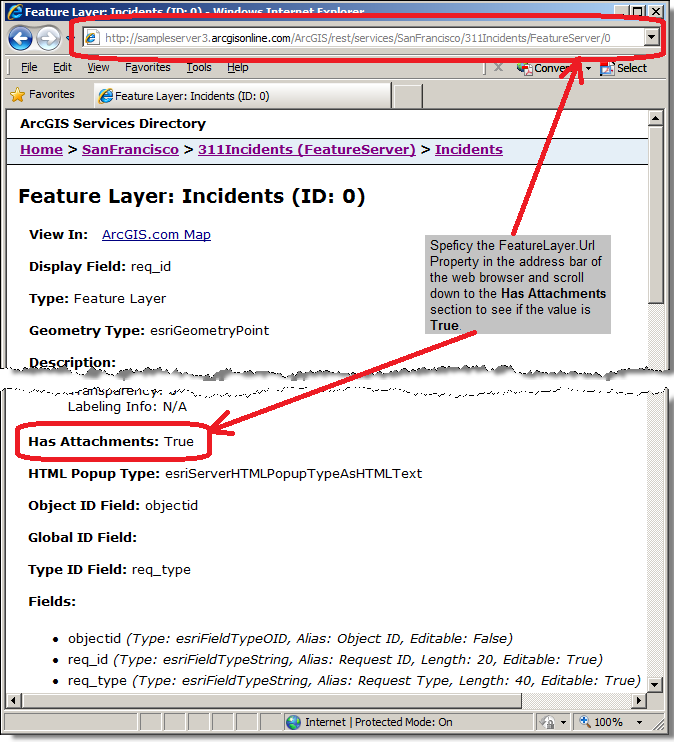
Additionally, developers can programmatically discover if a FeatureLayer Has Attachments by using the FeatureLayer.LayerInfo Property to return an ESRI.ArcGIS.Client.FeatureService.FeatureLayerInfo Class. Use the ESRI.ArcGIS.Client.FeatureService.FeatureLayerInfo.HasAttachments Property to obtain a Boolean value if attachments are enabled for the FeatureService. A code example is provided for programmatically discovering if a FeatureLayer Has Attachments in the AttachmentEditor.FeatureLayer Property documentation.
If the AttachmentEditor.FeatureLayer Property is not set to a valid ArcGIS Server FeatureServer service with attachments enabled, a Visual Studio NotSupportedException error containing syntax similar to the following will occur: "Layer does not support attachments" or "Layer does not support adding attachments". It should be noted the FeatureLayer.Url Property of an ArcGIS Server MapServer service looks very similar to FeatureServer and might even display a Has Attributes value equal True when viewed in a web browser but this should not be used for the AttachmentEditor Control. A valid FeatureServer will have the words "FeatureServer" in the URL, not "MapServer" which will cause errors.
It is the AttachmentEditor.GraphicSource Property that causes the AttachmentEditor to make a call to the ArcGIS Server FeatureServer to see if any existing attachments are associated with a specific Graphic in the FeatureLayer stored in the backend database. If any attachments are found to be associated with a Graphic in the FeatureLayer, they will be listed in the ListBox portion of the control. For each attachment that is listed in the ListBox of the AttachmentEditor, users can click on the hyperlink with the attachment filename to use the Microsoft File Download dialog to save the file on the local machine. Attachments listed in the ListBox can also be deleted from the backend ArcGIS Server FeatureService by clicking the red X next to the attachment name.
When a Graphic is associated with the AttachmentEditor.GraphicSource Property the Add button of the AttachmentEditor becomes enabled. The Add button allows users on the client machine to upload files on the local computer to the ArcGIS Server FeatureServer backend database via the Microsoft OpenFileDialog dialog. The AttachmentEditor.Filter, AttachmentEditor.FilterIndex, and AttachmentEditor.Multiselect Properties control the behavior of the Microsoft OpenFileDialog, see the following screen shot:
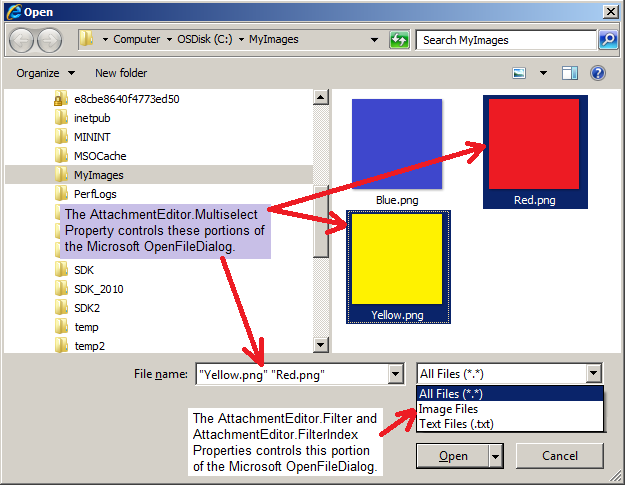
System.Object
System.Windows.Threading.DispatcherObject
System.Windows.DependencyObject
System.Windows.Media.Visual
System.Windows.UIElement
System.Windows.FrameworkElement
System.Windows.Controls.Control
ESRI.ArcGIS.Client.Toolkit.AttachmentEditor
Target Platforms: Windows XP Professional, Windows Server 2003 family, Windows Vista, Windows Server 2008 family