Visual Basic (Declaration) | |
---|---|
Public Property LayerDefinitions As ObservableCollection(Of LayerDefinition) |
C# | |
---|---|
public ObservableCollection<LayerDefinition> LayerDefinitions {get; set;} |
How to use: The following screenshot corresponds example code in this document where various LayerDefinitions are applied to an ArcGISDynamicMapServiceLayer showing earthquakes since 1970. Copy the XAML and/or desired code-behind into your project and adjust accordingly.
The XAML code in this example showing how to use a LayerDefinition can be used independently of the code-behind (C# or VB.NET) to demonstrate the functionality. In order to use the code-behind to show how a LayerDefinition works, make sure to comment out the usage of the LayerDefinition syntax in XAML.
XAML | ![]() |
---|---|
<StackPanel Name="StackPanel1" Height="400" Width="400" Margin="0,0,0,0" HorizontalAlignment="Left" VerticalAlignment="Top" > <esri:Map Background="White" Name="Map1" Height="200" Width="400"> <!-- Set the LayerDefinition for the ArcGISDynamicMapServiceLayer. --> <!-- By default no LayerDefinition is set unless explicitly set on the server. --> <esri:ArcGISDynamicMapServiceLayer Url="http://sampleserver3.arcgisonline.com/ArcGIS/rest/services/Earthquakes/Since_1970/MapServer" /> <esri:ArcGISDynamicMapServiceLayer.LayerDefinitions> <!-- LayerID="0" is the Earthquakes1970 layer. The Definition only displays earth quakes that have a Magnitude greater than 6. The field Magnitude is of type esriFieldTypeDouble. --> <esri:LayerDefinition LayerID="0" Definition="Magnitude > 6" /> <!-- LayerID="0" is the Earthquakes1970 layer. The Definition only displays earth quakes that have a Magnitude greater than 3 and less than 6. The field Magnitude is of type esriFieldTypeDouble. --> <!-- <esri:LayerDefinition LayerID="0" Definition="Magnitude > 3 AND Magnitude < 6" /> --> <!-- Another example where the Name of the earth quake event is to contains the letters 'CHINA'. Note: the Definition is case sensitive. The field Name is of type esriFieldTypeString. --> <!-- <esri:LayerDefinition LayerID="0" Definition="Name LIKE 'CHINA'" /> --> <!-- Another example where the Name of the earth quake event is exactly matches the letters 'VENEZUELA'. Note: the Definition is case sensitive. The field Name is of type esriFieldTypeString. --> <!-- <esri:LayerDefinition LayerID="0" Definition="Name = 'VENEZUELA'" /> --> <!-- Another example where the earth quake events are displayed if they occured after January 15, 2000. The field Date_ is of type esriFieldTypeDate. --> <!-- <esri:LayerDefinition LayerID="0" Definition="Date_ > DATE '1/15/2000'" />--> </esri:ArcGISDynamicMapServiceLayer.LayerDefinitions> </esri:ArcGISDynamicMapServiceLayer> </esri:Map> <!-- LayerDefinitions Property (Read) --> <TextBlock Height="23" Name="TextBlock_LayerDefinitions" Text="{Binding ElementName=Map1, Path=Layers[0].LayerDefinitions[0].Definition}" /> </StackPanel> |
C# | ![]() |
---|---|
private void ArcGISDynamicMapServiceLayer_Initialized(object sender, System.EventArgs e) { // The myArcGISDynamicMapServiceLayer (an ArcGISDynamicServiceLayer object) and TextBlock_LayerDefinitions // (a TextBlock object) were defined previously in the XAML or code-behind. // Get the first layer in the LayerInfo collection. ArcGISDynamicMapServiceLayer myArcGISDynamicMapServiceLayer = (ArcGISDynamicMapServiceLayer)Map1.Layers[0]; // LayerDefinition (Read/Write) // This is how to set (i.e. Write) a LayerDefinition. // -------------------------------------------------- // Set the .LayerDefinition for the .LayerID = 0 (the Earthquakes1970 layer). By default no LayerDefinition // is set unless explicitly set on the server. ESRI.ArcGIS.Client.LayerDefinition myDefinition = new ESRI.ArcGIS.Client.LayerDefinition(); myDefinition.LayerID = 0; // The Definition only displays earth quakes that have a Magnitude greater than 6. // The field Magnitude is of type esriFieldTypeDouble. myDefinition.Definition = "Magnitude > 6"; // The Definition only displays earth quakes that have a Magnitude greater than 3 and less that 6. // The field Magnitude is of type esriFieldTypeDouble. // myDefinition.Definition = "Magnitude > 3 AND Magnitude < 6"; // Another example where the Name of the earth quake event is to contains the letters 'CHINA'. // Note: the Definition is case sensitive. The field Name is of type esriFieldTypeString. //myDefinition.Definition = "Name LIKE 'CHINA'"; // Another example where the Name of the earth quake event is exactly matches the letters 'VENEZUELA'. // Note: the Definition is case sensitive. The field Name is of type esriFieldTypeString. //myDefinition.Definition = "Name = 'VENEZUELA'"; // Another example where the earth quake events are displayed if they occured after January 15, 2000. // The field Date_ is of type esriFieldTypeDate. //myDefinition.Definition = "Date_ > DATE '1/15/2000'"; // Create an ObservableCollection and add the .Definition to it. System.Collections.ObjectModel.ObservableCollection<LayerDefinition> myObservableCollection2 = new System.Collections.ObjectModel.ObservableCollection<LayerDefinition>(); myObservableCollection2.Add(myDefinition); // Apply the custom LayerDefinition myArcGISDynamicMapServiceLayer.LayerDefinitions = myObservableCollection2; // This is how to get (i.e. Read) a LayerDefiniton. // The TextBlock_LayerDefinitions (a TextBlock object) was previously set in XAML or code-behind. // ---------------------------------------------------------------------------------------------- // Get the existing LayerDefinitions collection. System.Collections.ObjectModel.ObservableCollection<LayerDefinition> myObservableCollection = myArcGISDynamicMapServiceLayer.LayerDefinitions; if (myObservableCollection.Count > 0) { // Get the first LayerDefinition ESRI.ArcGIS.Client.LayerDefinition myLayerDefinition = myObservableCollection[0]; // Display some textual information about the LayerDefinition. TextBlock_LayerDefinitions.Text = "For Layer: " + myLayerDefinition.LayerID.ToString() + ". The Defintion is: " + myLayerDefinition.Definition; } else { TextBlock_LayerDefinitions.Text = "[NO LayerDefinitions SET]"; } } |
VB.NET | ![]() |
---|---|
Private Sub ArcGISDynamicMapServiceLayer_Initialized(ByVal sender As System.Object, ByVal e As System.EventArgs) ' The myArcGISDynamicMapServiceLayer (an ArcGISDynamicServiceLayer object) and TextBlock_LayerDefinitions ' (a TextBlock object) were defined previously in the XAML or code-behind. ' Get the first layer in the LayerInfo collection. Dim myArcGISDynamicMapServiceLayer As ArcGISDynamicMapServiceLayer = Map1.Layers.Item(0) ' LayerDefinition (Read/Write) ' This is how to set (i.e. Write) a LayerDefinition. ' -------------------------------------------------- ' Set the .LayerDefinition for the .LayerID = 0 (the Earthquakes1970 layer). By default no LayerDefinition ' is set unless explicitly set on the server. Dim myDefinition As New ESRI.ArcGIS.Client.LayerDefinition myDefinition.LayerID = 0 ' The Definition only displays earth quakes that have a Magnitude greater than 6. ' The field Magnitude is of type esriFieldTypeDouble. myDefinition.Definition = "Magnitude > 6" ' The Definition only displays earth quakes that have a Magnitude greater than 3 and less than 6. ' The field Magnitude is of type esriFieldTypeDouble. 'myDefinition.Definition = "Magnitude > 3 AND Magnitude < 6" ' Another example where the Name of the earth quake event is to contains the letters 'CHINA'. ' Note: the Definition is case sensitive. The field Name is of type esriFieldTypeString. 'myDefinition.Definition = "Name LIKE 'CHINA'" ' Another example where the Name of the earth quake event is exactly matches the letters 'VENEZUELA'. ' Note: the Definition is case sensitive. The field Name is of type esriFieldTypeString. 'myDefinition.Definition = "Name = 'VENEZUELA'" ' Another example where the earth quake events are displayed if they occured after January 15, 2000. ' The field Date_ is of type esriFieldTypeDate. 'myDefinition.Definition = "Date_ > DATE '1/15/2000'" ' Create an ObservableCollection and add the .Definition to it. Dim myObservableCollection2 As New System.Collections.ObjectModel.ObservableCollection(Of LayerDefinition) myObservableCollection2.Add(myDefinition) ' Apply the custom LayerDefinition myArcGISDynamicMapServiceLayer.LayerDefinitions = myObservableCollection2 ' This is how to get (i.e. Read) a LayerDefiniton. ' The TextBlock_LayerDefinitions (a TextBlock object) was previously set in XAML or code-behind. ' ---------------------------------------------------------------------------------------------- ' Get the existing LayerDefinitions collection. Dim myObservableCollection As System.Collections.ObjectModel.ObservableCollection(Of LayerDefinition) = _ myArcGISDynamicMapServiceLayer.LayerDefinitions If myObservableCollection.Count > 0 Then ' Get the first LayerDefinition Dim myLayerDefinition As LayerDefinition = myObservableCollection.Item(0) ' Display some textual information about the LayerDefinition. TextBlock_LayerDefinitions.Text = "For Layer: " + myLayerDefinition.LayerID.ToString + _ ". The Defintion is: " + myLayerDefinition.Definition Else TextBlock_LayerDefinitions.Text = "[NO LayerDefinitions SET]" End If End Sub |
Definition expressions for particular LayerID in the LayerDefinition class that are currently not visible will be ignored by the server.
When the LayerDefinitions Property is not set, all features are returned.
A LayerDefinition of 1=1 returns all features.
For a LayerDefinition that contains an apostrophe embedeed within an esriFieldTypeString, escape the apostrophe with a second apostrophe. Example: Name = 'Billy ''O Donald'.
For a LayerDefinition that is based upon a numeric value (i.e. esriFieldTypeDouble, esriFieldTypeInt, etc.) when performing a 'less than' (i.e. the < character) operation in XAML, make sure and escape the syntax with the characters <. Example: Cost < 100.
For ArcGISDynamicMapServiceLayers that are served up by ArcGIS Server 10 and above, it is possible to use Time as part of a DATE LayerDefintion. Example: Birthday > DATE '1/15/2008 18:30:00' or Observation1 = DATE '12/27/2008 03:15:00'. For ArcGISDynamicMapServiceLayers that are served up by ArcGIS 9.31 and earlier, Time portions of DATE LayerDefinitions are not supported. Use the following syntax instead: Birthday > DATE '1/15/2008' or Observation1 = DATE '12/27/2008'.
TIP: One way to speed up development time in determining the correct LayerDefinition syntax for the ObservableCollections(Of LayerDefinitions) is to use Query functionality for the web service in a web browser. The way you do this is via the following steps:
1. Copy the ArcGISDynamicMapServiceLayer.URL property into the address bar of the web browser and then click on the hyperlink for the specific LayerID you want to perform a Query on. See the following screen shot:
2. For the specific ArcGISDynamicMapServiceLayer, scroll to the bottom of the web page and click on the Query hyperlink. See the following screen shot:
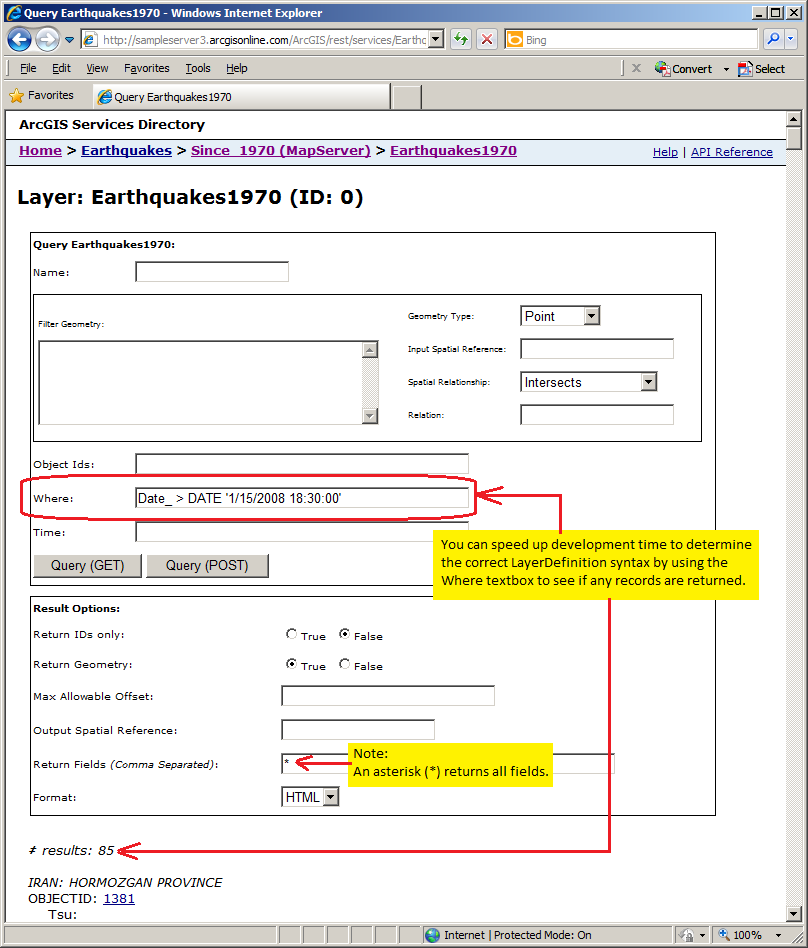
Target Platforms: Windows XP Professional, Windows Server 2003 family, Windows Vista, Windows Server 2008 family