A raster
dataset contains one or more layers called bands. For example, a color image has three bands (red, green, and blue) while a digital elevation model (DEM) has one band
(holding elevation values), and a multispectral image may have many bands.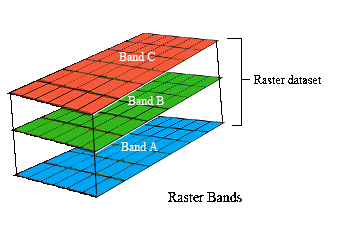
Each raster
band contains the actual cell values, as well as some key properties,
such as:
- Statistics: minimum, maximum, and mean cell values
- Histogram of cell values
- Default color map for raster display (optional)
Examples of a 1 band raster are
- Elevation Data
- Grayscale aerial imagery
- Scanned maps/documents
Examples of 3 band raster are
- Most color TIFFs, IMGS or MrSIDSs
- Remotely Sensed/Spectral data
In the Raster Java API, a band in a raster band is represented by the SeRasterBand
class. As well the SeRasterAttr object stores band metadata such as number of
bands and width of bands, while the actual bands can be retrieved in the form of
a SeRasterBand array from SeRaster using the SeRaster.getBands() method.
Prior to retrieving bands from SeRaster, it is necessary to obtain all raster
information from the server. This is accomplished by a call to the
SeRaster.getInfoById(lastInsertID) method. Consequently, bands can be retrieved
by calling the SeRaster.getBands() method.
From the Raster C API, a band in a raster is accessed through the SE_RASBANDINFO
structure. As well the SE_RASTERATTR structure stores band metadata such as
number of bands and width of bands, while the actual bands can be retrieved in
the form of a SE_RASBANDINFO array from SE_RASTERATTR using the
SE_rasterattr_get_rasterband_info_list() method.
See also
To retrieve raster data such as bands, the user must first obtain all raster information from the server and then retrieve band information in the
form of a raster band array.
SE_CONNECTION
connection;
CHAR
table[SE_MAX_TABLE_LEN],
raster_column[SE_MAX_COLUMN_LEN];
LONG
object_id;
SE_RASBANDINFO *t_rasterbands,*rasterbands;
LONG
*number_of_bands
SE_RASTERATTR raster_attrib;
SE_STREAM stream;
SE_QUERYINFO
queryinfo;
CHAR
**tables,
**columns,
whereclause[32],
raster_column[SE_MAX_COLUMN_LEN];
LONG
rc;
SHORT
raster_ind,i;
/*
create the connection to the ArcSDE geodatabase */
rc =
SE_connection_create(“myserver”,
”myinstance”,
”mydatabase”,
”myuser”,
”mypassword”,
&error,
&connection);
check_error (rc, NULL, NULL, "SE_rasterattr_create");
strcpy(table,”my_table”);
strcpy(raster_column,”myraster”);
/*
Initialize SE_RASATTR structure */
rc =
SE_rasterattr_create(&raster_attrib,FALSE);
check_error
(rc, NULL, NULL, "SE_rasterattr_create");
rc =
SE_stream_create(connection,&stream);
check_error (rc, connection, NULL, "SE_stream_create");
/*
Create the queryinfo structure */
rc =
SE_queryinfo_create (&queryinfo);
check_error (rc, connection, NULL, "SE_stream_create");
/* Add
the raster table to the query info structure */
tables
= (CHAR **)malloc(sizeof(CHAR*));
tables[0] = malloc((strlen(table) +1) * sizeof(CHAR));
strcpy(tables[0],table);
rc =
SE_queryinfo_set_tables(queryinfo,1,(const CHAR **)tables,NULL);
check_error (rc, connection, NULL, "SE_queryinfo_set_table");
free(tables);
/* Add
the raster table's columns to the query info structure */
columns
= (CHAR **)malloc(sizeof(CHAR*));
columns[0] = malloc((strlen(raster_column) +1) * sizeof(CHAR));
strcpy(columns[0],raster_column);
rc =
SE_queryinfo_set_columns (queryinfo,1,(const CHAR **)columns);
check_error (rc, connection, NULL, "SE_queryinfo_set_table");
free(columns);
sprintf(whereclause,"objectid = %d",object_id);
rc =
SE_queryinfo_set_where_clause(queryinfo,whereclause);
check_error (rc, NULL, NULL, "SE_queryinfo_set_whereclause");
/* Add
the queryinfo structure to the stream */
rc =
SE_stream_query_with_info (stream, queryinfo);
check_error (rc, NULL, stream, "SE_stream_query_with_info");
/* Free
the queryinfo, we are done with it. */
SE_queryinfo_free(queryinfo);
/* Bind
the output of the stream the raster column */
rc =
SE_stream_bind_output_column (stream,
1,
raster_attrib,
&raster_ind);
check_error (rc, NULL, stream, "SE_stream_bind_output_column");
/*
Execute the query */
rc =
SE_stream_execute (stream);
check_error (rc, NULL, stream, "SE_stream_execute");
while
(SE_SUCCESS == rc)
/* Fetch each record of the business table */
rc = SE_stream_fetch (stream);
if (SE_FINISHED != rc)
{
check_error (rc, NULL, stream, "SE_stream_fetch");
if(raster_ind==SE_IS_NOT_NULL_VALUE) {
rc = SE_rasterattr_get_rasterband_info_list (
raster_attrib, &t_rasterband,
number_of_bands); ; check_error (rc, NULL, NULL,
"SE_rasterattr_get_rasterband_info_list");
} }
}
}
*rasterbands =
(SE_RASBANDINFO *)malloc (sizeof(SE_RASBANDINFO)*(*number_of_bands));
for(i=0;i<(*number_of_bands);i++) {
rc = SE_rasbandinfo_create(&((*rasterbands)[i]));
check_error
(rc, NULL, stream, ret, "SE_rasbandinfo_create");
rc = SE_rasbandinfo_duplicate(t_rasterbands[i],(*rasterbands)[i]);
check_error (rc, NULL, stream, ret, "SE_rasbandinfo_duplicate");
}
SE_rasterattr_free(raster_attrib);
SE_queryinfo_free(queryinfo);
rc =
SE_stream_free(stream);
check_error (rc, NULL, NULL, "SE_stream_free");
SeObjectId lastInsertId = insert.lastInsertedRasterId();
SeRasterAttr attr = ......;
SeRaster raster = attr.getRasterInfo();
//obtain all raster information from the server
raster.getInfoById(lastInsertId);
SeRasterBand[] bands = raster.getBands();
for(int i = 0; i < bands.length; i++)
{
displayRasterBands(bands[i]);//display band attributes
}
//Clean Up: Delete column, table, close connection.
Tip: In second case, raster column attributes should be set after instantiating
the object.
|
A raster can contain multiple bands (for example, a multispectral image). A new band can be created and added to the raster using methods provided in SeRasterBand.
Variables that represent band attributes, such as bandNum and compressionType, can either be given fresh values or can be retrieved from corresponding SeRasterAttr
objects. For possible assignment values, please consult the Raster API doc.
SE_CONNECTION
connection;
SE_ERROR error;
CHAR
table[SE_MAX_TABLE_LEN];
LONG
sequence_nbr,
LONG
compression,
LONG
interpolation,
LONG
pixel_type
LONG rc;
SE_RASBANDINFO rasterband;
SE_RASCOLINFO rascol_info;
SE_RASTERINFO raster;
LONG rastercolumn_id;
LONG tile_width;
LONG tile_height;
LONG raster_width;
LONG raster_height;
LONG rasterband_id;
SE_ENVELOPE raster_env;
/*
Initialize the SE_RASBANDINFO structure */
rc =
SE_rasbandinfo_create (&rasterband);
check_error (rc, NULL, NULL, "SE_rasbandinfo_create");
/*
Initialize SE_RASCOLINFO structure */
rc =
SE_rascolinfo_create(&rascol_info);
check_error (rc, NULL, NULL, "SE_rascolinfo_create");
/*
Initialize SE_RASTERINFO structure */
rc =
SE_rasterinfo_create (&raster); check_error (rc, NULL, NULL, "SE_rasterinfo_create");
/*
Populate the SE_RASCOLINFO structure*/
rc =
SE_rastercolumn_get_info_by_name (connection,
table,
"raster",
rascol_info);
check_error (rc, connection, NULL, "SE_rastercolumn_get_info_by_name");
/* Get
the rastercolumn id */
rc =
SE_rascolinfo_get_id (rascol_info, &rastercolumn_id);
check_error (rc, NULL, NULL, "SE_rastercolumn_get_id");
/*
Populate the SE_RASTERINFO structure */
rc =
SE_raster_get_info_by_id (connection,
rastercolumn_id,
1,
raster);
check_error (rc, NULL, NULL, "SE_raster_get_info_by_id");
/*
Populate the SE_RASBANDINFO structure */
/* Set
the raster column id */
rc =
SE_rasbandinfo_set_rascol_id (rasterband, rastercolumn_id);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_rascol_id");
/* Set
the image compression */
rc =
SE_rasbandinfo_set_compression_type ( rasterband, compression);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_compression_type");
/* Set
pixel type */
rc =
SE_rasbandinfo_set_pixel_type (rasterband, pixel_type);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_pixel_type");
/* Set
the tile size */
tile_width = 64;
tile_height = 64;
rc =
SE_rasbandinfo_set_tile_size (rasterband, tile_width, tile_height);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_tile_size");
/* Set
the extent */
calc_extent(&raster_env,0,0,255,255);
rc =
SE_rasbandinfo_set_extent (rasterband, &raster_env);
check_error
(rc, NULL, NULL, "SE_rasbandinfo_set_extent");
/* Set
the band size */
raster_width = 256;
raster_height = 256;
rc =
SE_rasbandinfo_set_band_size
(rasterband,raster_width,raster_height);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_image_size");
/* Set
the maximum pyramid level */
rc =
SE_rasbandinfo_set_max_level (rasterband, 2, FALSE);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_max_level");
/* Set
the band name */
rc =
SE_rasbandinfo_set_band_name (rasterband, "An added band");
check_error (rc, NULL, NULL, "SE_rasterattr_set_max_level");
/* Set
the band sequence number */
rc =
SE_rasbandinfo_set_band_number (rasterband, sequence_nbr);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_band_number");
/* Add
a record to the SDE_BND_<N> table. */
rc =
SE_rasterband_create (connection, rasterband, &rasterband_id);
check_error (rc, connection, NULL, "SE_rasterband_create");
rc =
SE_rasterband_get_info_by_id( connection,
rastercolumn_id,
rasterband_id,
rasterband);
check_error (rc, connection, NULL, "SE_rasterband_get_info_by_id");
rc =
SE_raster_add_band (connection, raster, rasterband);
check_error (rc, NULL, NULL, "SE_rasterband_add_band");
SE_rasbandinfo_free(rasterband);
SE_rasterinfo_free(raster);
SE_rascolinfo_free(rascol_info);
Java CONTENT
|
In the following code, the above SeRasterBand, band1, is altered by giving it a different name, compression type, pixel type, and setting a function for calculating statistical data. A call to SeRasterBand.alter() (line 5) alters the band in the raster.
SE_CONNECTION
connection;
CHAR
table[SE_MAX_TABLE_LEN];
LONG objectid;
LONG rc,
band,
number_of_bands;
SE_RASBANDINFO *rasbandinfo_list;
LONG num_bins;
SE_BIN_FUNCTION_TYPE bin_function_type;
LONG
goal;
CHAR bandname[24];
/* fetch the raster bands for the raster */
get_rasterbands( connection,
table,
raster_id,
&rasbandinfo_list,
&number_of_bands);
for (band=0; band<number_of_bands; band++) {
num_bins = 0;
bin_function_type = SE_BIN_FUNCTION_AUTO;
/* Set the bin function to automatic complete with bin
table. */
rc = SE_rasbandinfo_set_bin_function ( rasbandinfo_list[band],
bin_function_type,
0,
NULL);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_bin_function");
sprintf(bandname,"band number %d\0",band+1);
rc = SE_rasbandinfo_set_band_name(rasbandinfo_list[band],bandname);
check_error (rc, NULL, NULL, "SE_rasbandinfo_set_band_name");
/* Add the statistics to the rasterband. */
rc = SE_rasterband_alter (connection, rasbandinfo_list[band]);
check_error (rc, connection, NULL, "SE_rasterband_alter");
}
for (band=0; band<number_of_bands; band++)
SE_rasbandinfo_free(rasbandinfo_list[band]);
free(rasbandinfo_list);
band1.setBandName("Band Altered")
band1.setCompressionType(compressionType);
band1.setPixelType(pixelType);
int numBanks = 3;
int numEntries = 256;
DataBufferByte dataBuffer = new DataBufferByte(numEntries, numBanks);
for (int elem = 0; elem < numEntries; elem++)
{
for (int bank = 0; bank < numBanks; bank++)
{
dataBuffer.setElem (bank, elem, (int) elem);
}
}
band1.setColorMap(SeRaster.SE_COLORMAP_RGB, dataBuffer);
band1.alter();
|
A band can be deleted or dropped by calling SeRasterBand's delete() or dropBand() methods respectively. The difference between these
two methods is that dropBand()
merely removes the band information from the object, with a place for the band still available inside the raster, and delete() completely
removes all traces of that particular band.
SE_CONNECTION
connection;
CHAR
table_name[SE_MAX_TABLE_LEN];
LONG
sequence_nbr;
LONG
rc;
SE_RASBANDINFO *rasbandinfo_list;
SE_RASCOLINFO rascol_info;
SE_RASTERINFO raster;
LONG rastercolumn_id;
LONG number_of_bands;
LONG band_number;
LONG band;
/* Initialize SE_RASCOLINFO structure */
rc =
SE_rascolinfo_create(&rascol_info);
check_error (rc, NULL, NULL, "SE_rascolinfo_create");
/* Initialize the SE_RASTERINFO structure */
rc =
SE_rasterinfo_create (&raster);
check_error (rc, NULL, NULL, "SE_rasterinfo_create");
/* Populate the SE_RASCOLINFO structure*/
rc =
SE_rastercolumn_get_info_by_name (connection,
table,
"raster",
rascol_info);
check_error (rc, connection, NULL, "SE_rastercolumn_get_info_by_name");
/* Get the rastercolumn id */
rc =
SE_rascolinfo_get_id (rascol_info, &rastercolumn_id);
check_error (rc, NULL, NULL, "SE_rastercolumn_get_id");
/* Populate the SE_RASTERINFO structure */
rc =
SE_raster_get_info_by_id (connection,
rastercolumn_id,
1,
raster);
check_error (rc, connection, NULL, "SE_raster_get_info_by_id");
rc =
SE_raster_get_bands ( connection,
raster,
&rasbandinfo_list,
&number_of_bands);
check_error (rc, connection, NULL, "SE_raster_get_bands");
for (band=0;band<number_of_bands;band++) {
rc = SE_rasbandinfo_get_band_number(rasbandinfo_list[band],
check_error
(rc, connection, NULL,
"SE_rasbandinfo_get_band_number");
if (band_number == sequence_nbr) {
rc = SE_rasterband_delete (connection,
rasbandinfo_list[band]);
check_error (rc, NULL, NULL, "SE_rasterband_delete");
}
}
/* Free the structures */
SE_rasterband_free_info_list(number_of_bands,rasbandinfo_list);
SE_rascolinfo_free(rascol_info);
SeRasterBand[] bands2 = raster.getBands();
System.out.println("Number of Bands: " + bands2.length);
//Dropping band
raster.dropBand(bands2[0]);
//Deleting band
bands2[1].delete();
bands2 = null;
bands2 = raster.getBands();
System.out.println("Number of Bands: " + bands2.length); Tip: After SeRaster.dropBand() is executed, the value of the Raster_ID
column, which is the foreign key to the Raster table, becomes negative
|
Descriptive text
if(SE_rasbandinfo_has_colormap (rasband_info)){
rc = SE_rasbandinfo_get_colormap( rasband_info,
&colormap_type,
&colormap_data_type,
&num_cm_entries,
(void**)&colormap_data);
check_error (rc, NULL, NULL, "SE_rasbandinfo_get_colormap");
}
SeRasterBand.SeRasterBandColorMap
|
Descriptive text
if (SE_rasbandinfo_has_stats
(rasband_info)) {
/* Display the histogram */
rc = SE_rasbandinfo_get_stats_histogram (rasband_info,
&bin_function_type,
&num_bins,
&bin_table,
&histogram);
check_error
(rc, NULL, NULL, "SE_rasbandinfo_get_stats_histogram");
switch (bin_function_type) {
case SE_BIN_FUNCTION_NONE:
printf("Bin function type is NONE\n");
break ;
case SE_BIN_FUNCTION_AUTO:
printf("Bin function type is AUTO\n");
break ;
case SE_BIN_FUNCTION_DIRECT:
printf("Bin function type is DIRECT\n");
break ;
case SE_BIN_FUNCTION_LINEAR:
printf("Bin function type is LINEAR\n");
break ;
case SE_BIN_FUNCTION_LOGARITHM:
printf("Bin function type is LOGARITHM\n");
break ;
case SE_BIN_FUNCTION_EXPLICIT:
printf("Bin function type is EXPLICIT\n");
break ;
default :
printf("Unknown bin function type\n");
}
printf("Raster band number of bins: %d\n",num_bins);
if (bin_table)
{
for (h=0;h<=num_bins;h++)
printf("Bin table element %d is: %lf\n",h,bin_table[h]);
}
str_val = (CHAR*)malloc(20*num_bins* sizeof (CHAR));
strcpy(str_val, "");
/* Display the minimum statistic */
rc = SE_rasbandinfo_get_stats_min (rasband_info, &stats_min);
check_error(rc, NULL, NULL, "SE_rasbandinfo_get_stats_min");
printf("Raster band statistical minimum: %lf\n",stats_min);
/* Display the maximum statistic */
rc
= SE_rasbandinfo_get_stats_max (rasband_info, &stats_max);
check_error (rc, NULL, NULL,
"SE_rasbandinfo_get_stats_max");
printf("Raster band statistical
maximum: %lf\n",stats_max);
/* Display the mean */
rc
= SE_rasbandinfo_get_stats_mean (rasband_info, &mean);
check_error (rc, NULL, NULL, "SE_rasbandinfo_get_stats_mean");
/* Display the standard deviation */
rc
= SE_rasbandinfo_get_stats_stddev (rasband_info, &stddev);
check_error (rc, NULL, NULL, "SE_rasbandinfo_get_stats_stddev");
printf("Raster
band standard deviation: %lf\n\n",stddev);
SeRasterBand.SeRasterBandStats
|
|