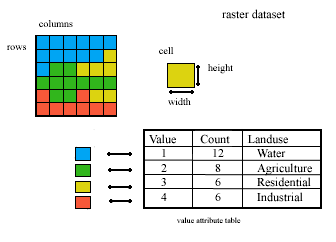
In ArcSDE, the raster is represented by the Raster API object and meta-information
regarding raster bands, pixel types, etc is handled by the Raster Attribute
object.
When a raster is created, ArcSDE adds a row to the RasterColumn
table and creates related records in the Raster Band (SDE_BND_),
Raster Block(SDE_BLK_), and Raster Auxiliary (SDE_AUX_)
tables. The Raster
Attribute object (SeRasterAttr in Java and SE_RASTERATTR structure in
C API) contains information about raster attributes such as number of
tiles, size of tiles, number of bands in raster, band width, interpolation,
maximum level, and the type and depth of pixels.
Creating a Raster Attribute object
/* A valid SE_RASTERATTR structure must be created before using
any other function that requires an SE_RASTERATTR pointer. The SE_RASTERATTR
structure contains information about raster attributes and is used
to create a valid raster. */ LONG rc, max_level, raster_width = 256, raster_height = 256, tileWidth
= 64, tileHeight = 64, number_of_bands = 3, num_colormap_entries, bits_per_pixel,bytes;
SE_RASTERATTR raster_attrib;
SE_STREAM stream;
SE_ENVELOPE rect;
SE_COLORMAP_TYPE *colormap_type;
SE_COLORMAP_DATA_TYPE *colormap_data_type;
UCHAR *colormap_data;
BOOL bitmask, scanline;
/* Create the SE_RASTERATTR raster attribute info structure */
rc = SE_rasterattr_create (&raster_attrib, FALSE);
rc = SE_rasterattr_set_pixel_type(raster_attrib, SE_PIXEL_TYPE_8BIT_U);
/*Create an 8-bit RGB Colormap array "colormap_data" and use
in following function call*/
rc = SE_rasterattr_set_colormap (*raster_attrib, SE_COLORMAP_RGB, SE_COLORMAP_DATA_BYTE,
256, (void *)colormap_data);
rc = SE_rasterattr_set_compression_type(*raster_attrib, SE_COMPRESSION_LZ77);
rc = SE_rasterattr_set_image_size (*raster_attrib, raster_width, raster_height,
number_of_bands);
rc = SE_rasterattr_set_mask_mode (*raster_attrib, bitmask);
rc = SE_rasterattr_set_mosaic_mode (*raster_attrib, FALSE);
rc = SE_rasterattr_set_pyramid_info (*raster_attrib, max_level, FALSE,
interpolation);
rc = SE_rasterattr_set_pixel_type (*raster_attrib,pixel_type);
rc = SE_rasterattr_set_tile_size (*raster_attrib, tileWidth, tileHeight);
rc = SE_rasterattr_set_pyramid_info(*raster_attrib, max_level, FALSE, SE_INTERPOLATION_BILINEAR);
/* Set min and max for the rectangle and generate shape */
rect.minx = minx;
rect.miny = miny;
rect.maxx = maxx;
rect.maxy = maxy;
ret = SE_rasterattr_set_extent(*raster_attrib, &rect);
/*The callback function determines how SE_stream_execute will enter the
image pixel data into the raster column*/
ret = SE_rasterattr_set_callback(*raster_attrib, raster_callback_function(),
NULL);
//Bind the address of our input variables and indicators
ret = SE_stream_bind_input_column(stream, 4, raster_attrib, ?Null);
// Execute
ret = SE_stream_execute (stream);
// Free resources
ret = SE_stream_free (stream);
SE_rasterattr_free (*raster_attrib);
/*An SeRasterAttr object has to be created first and inserted into the database.
The SeRaster object is then retrieved from the SeRasterAttr object using the SeRasterAttr.getRasterInfo() method. Thus, creation of
SeRasterAttr is a pre-requisite for creating a SeRaster.*/ BufferedImage image = ..........;
int mBufImageType = image.getType();
int mBufImageWidth = image.getWidth();
int mBufImageHeight = image.getHeight();
boolean inputMode = true;
boolean skipLevelOne = false;
boolean maskMode = true;
int bandWidth = mBufImageWidth;
int bandHeight = mBufImageHeight;
int numBands = 3;
int tileWidth = bandWidth >> 3;
int tileHeight = bandHeight >> 3;
int pixelType = SeRaster.SE_PIXEL_TYPE_16BIT_U;
int compressTyp = SeRaster.SE_COMPRESSION_NONE;
int maxLevel = 3;
int interpolation = SeRaster.SE_INTERPOLATION_NONE;
int maskType = BufferedImageData.MASK_ALL_ON;
/**
SeRasterAttr object can be set in one of two modes:
TRUE - For load and insert operation AND
FALSE = For query and update operation.
*/
SeRasterAttr attr = new SeRasterAttr(inputMode);
attr.setImageSize(bandWidth, bandHeight, numBands);
attr.setTileSize(tileWidth, tileHeight);
attr.setPixelType(pixelType);
attr.setCompressionType(compressType);
attr.setPyramidInfo(maxLevel, skipLevelOne, interpolation);
attr.setMaskMode(maskMode);
SeExtent extent = new SeExtent(0, 0, bandWidth, bandHeight);
attr.setExtent(extent);
//Set callback func
Object userContext = null;
RasterCallBackImpl myRasCallBack = new RasterCallBackImpl(attr, maskType,
image);
attr.setRasterCallBack(myRasCallBack, userContext);
|
Constructing a Raster object from the Raster Attribute object or from the Raster Column ID.
C API Code
//Retrieve attr from table
SeSqlConstruct sqlCons = new SeSqlConstruct(tableName);
SeQuery query = new SeQuery(conn, columns, sqlCons);
query.prepareQuery();
query.execute();
SeRow row = query.fetch();
SeRasterAttr attr = row.getRaster(rastercolNum);
SeRaster raster = attr.getRasterInfo();
OR
SeConnection conn = ........;
SeRasterColumn rasterLayer = ........;
SeObjectId rasColId = rasterLayer.getID();
SeRaster raster = new SeRaster(conn, rasColId);
|
|